The Rational Agent
- Has a utility function
- Maximizes expected utility
- Sensors: perceives environment
- Actuators: influences environment
What is in between sensors and actuators?
The agent function.
Reflex Agent
- Very basic form of agent function
- Percept \(\rightarrow\) Action lookup table
- Good for simple games
- Needs entire state space in table
State Space Size
- Tic-tac-toe: \(10^3\)
- Checkers: \(10^{20}\)
- Chess: \(10^{44}\)
- Go: \(10^{170}\)
- Self-driving car: ?
- Pacman?
- How could you estimate it?
In Practice
- Environment
- Perception
- Action
- Measure/Reward
Search
- Fully-observed problem
- Deterministic actions and state
- Well defined start and goal
Not Search
- Uncertainty
- Adversary
- Cooperation
- Continuous state
Search Problem
Search problem includes:
- Start State
- State Space
- State Transitions
- Goal Test
State Space:
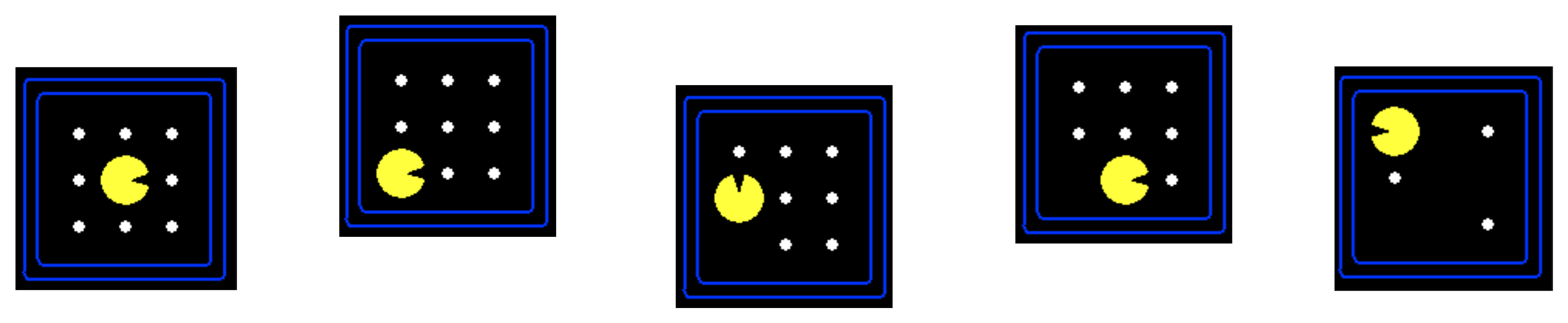
Actions & Successor States:
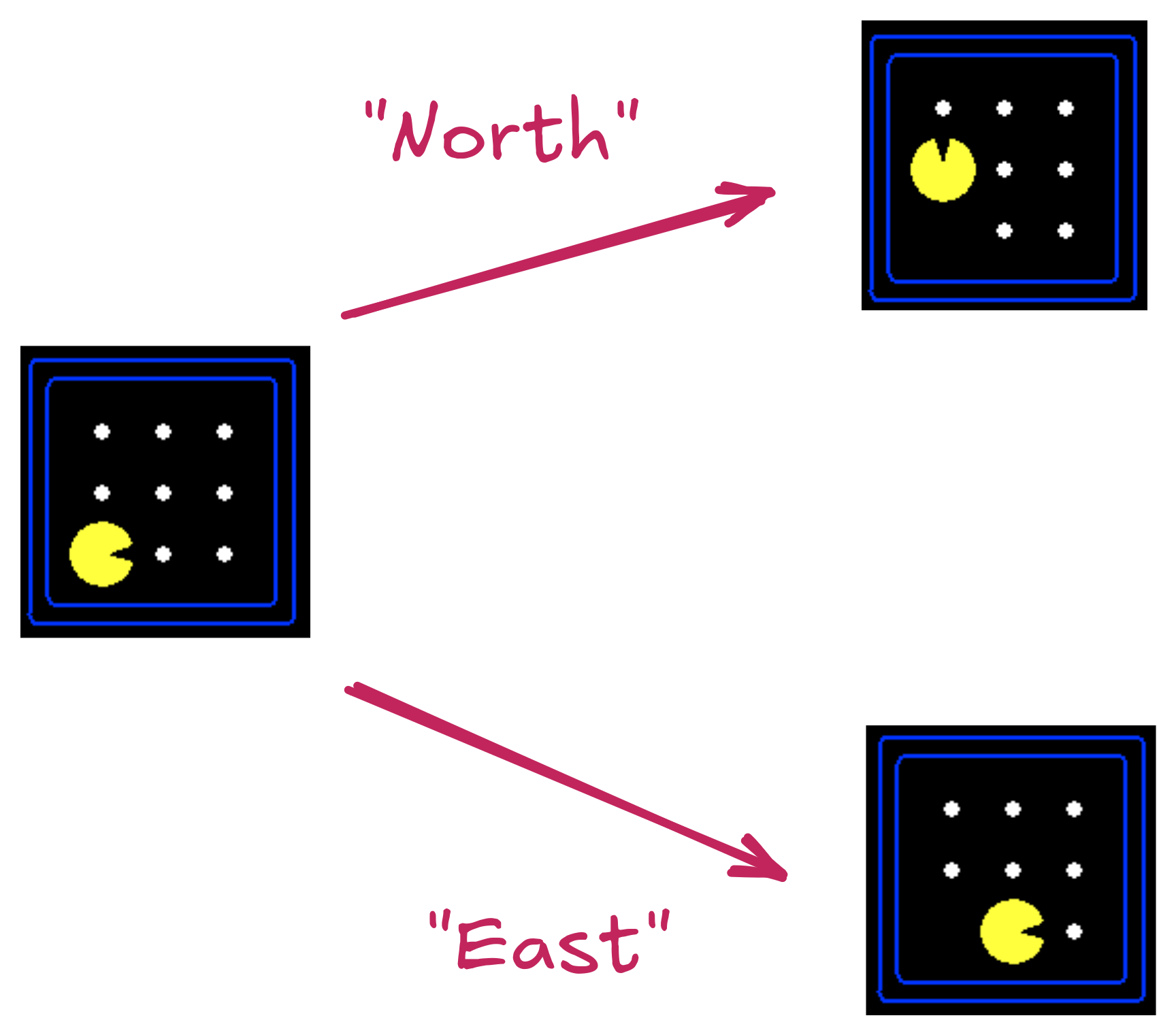
State Space
State Space Graph
How To Solve It
Given:
- Starting node
- Goal test
- Expansion
Do:
- Expand nodes from start
- Test each new node for goal
- Expand new nodes
- If nothing left to expand, failure
Queues & Searches
- Priority Queues
- Best-First Search
- Uniform-Cost Search1
- FIFO Queues
- LIFO Queues2
Search Features
- Completeness
- If there is a solution, will we find it?
- Optimality
- Will we find the best solution?
- Time complexity
- Memory complexity
Heuristics
heuristic - adj - Serving to discover or find out.1
- We know things about the problem
- These things are external to the graph/tree structure
- We could model the problem differently
- We can use the information directly
Choosing Heuristics
- Admissibility
- Never overestimates cost from \(n\) to goal
- Cost-optimal!
- Consistency
- \(h(n) \leq c(n, a, n') + h(n')\)
- \(n'\) successors of \(n\)
- \(c(n, a, n')\) cost from \(n\) to \(n'\) given action \(a\)
Weighted A* Search
- Greedy: \(f(n) = h(n)\)
- A*: \(f(n) = h(n) + g(n)\)
- Uniform-Cost Search: \(f(n) = g(n)\)
- Weighted A* Search: \(f(n) = W\cdot h(n) + g(n)\)
Iterative-Deepening A* Search
“IDA*” Search
- Similar to Iterative Deepening with Depth-First Search
- DFS uses depth cutoff
- IDA* uses \(h(n) + g(n)\) cutoff with DFS
- Once cutoff breached, new cutoff:
- Typically next-largest \(h(n) + g(n)\)
- \(O(b^m)\) time complexity 😔
- \(O(d)\) space complexity1 😌
Beam Search
Best-First Search:
- Frontier is all expanded nodes
Beam Search:
- \(k\) “best” nodes are kept on frontier
- Alt: all nodes within \(\delta\) of best node
- Not Optimal
- Not Complete
Where Do Heuristics Come From?
- Intuition
- Relaxation
- The problem is constrained
- Remove the constraint
- Pre-computation
- Learning
Local Search
Uninformed/Informed Search:
- Known start, known goal
- Search for optimal path
Local Search:
- “Start” is irrelevant
- Goal is not known
- But we know it when we see it
- Search for goal
Objective Function
- Do you know what you want?
- Can you express it mathematically?
- A single value
- More is better
- Objective function: a function of state
Hill-Climbing
- Objective function
- State space mapping
Hazards:
- Local maxima
- Plateaus
- Ridges
Variations
- Sideways moves
- Stochastic moves
- Random restarts
- If at first you don’t succeed,
you fail try again!
- Complete 😌
Simulated Annealing
- Search begins with high “temperature”
- Temperature decreases during search
- Next state selected randomly
- Improvements always accepted
- Non-improvements rejected stochastically
- Higher temperature, less rejection
- “Worse” result, more rejection
Local Beam Search
Recall:
- Beam search keeps track of \(k\) “best” branches
Local Beam Search:
- Hill climbing search, keeping track of \(k\) successors
Gradient Descent
- Minimize loss instead of climb hill
Consider:
- One state variable, \(x\)
- Objective function \(f(x)\)
- How do we minimize \(f(x)\) ?
- Is there a closed form \(\frac{d}{dx}\) ?
Gradient Descent
Multivariate \(\vec{x} = x_0, x_1, ...\)
Instead of derivative, gradient:
\(\nabla f(\vec{x}) = \left[ \frac{\partial f}{\partial x_0}, \frac{\partial f}{\partial x_1}, ...\right]\)
“Locally” descend gradient:
\(\vec{x} \gets \vec{x} + \alpha \nabla f(\vec{x})\)
I will not ask you to take a derivative on the exam.
Adversity
So far:
- The world does not care about us
- This is a simplifying assumption!
Reality:
The world does not care us
It wants things for “itself”
We don’t want the same things
The Adversary
One extreme:
- Single adversary
- Adversary wants the exact opposite from us
- If adversary “wins,” we lose 😐
Other extreme:
- An entire world of agents with different values
- They might want some things similar to us
- “Economics” 😐
Simple Games: Max and Min
- Two players want the opposite of each other
- State takes into account both agents
- Actions depend on whose turn it is
Minimax
- Initial state \(s_0\)
- Actions(\(s\)) and To-move(\(s\))
- Result(\(s, a\))
- Is-Terminal(\(s\))
- Utility(\(s, p\))
More Than Two Players
- Two players, two values: \(v_A, v_B\)
- Zero-sum: \(v_A = -v_B\)
- Only one value needs to be explicitly represented
- \(> 2\) players:
- \(v_A, v_B, v_C ...\)
- Value scalar becomes \(\vec{v}\)
Minimax Efficiency
Pruning removes the need to explore the full tree.
- Max and Min nodes alternate
- Once one value has been found, we can eliminate parts of search
- Lower values, for Max
- Higher values, for Min
- Remember highest value (\(\alpha\)) for Max
- Remember lowest value (\(\beta\)) for Min
Heuristics 😌
- In practice, trees are far too deep to completely search
- Heuristic: replace utility with evaluation function
- Better than losing, worse than winning
- Represents chance of winning
- Chance? 🎲🎲
- Even in deterministic games
- Why?
Solving Non-Deterministic Games
Previously: Max and Min alternate turns
Now:
Constraint Satisfaction
- Express problem in terms of state variables
- Constrain state variables
- Begin with all variables unassigned
- Progressively assign values to variables
- Assignment of values to state variables that “works:” solution
Constraint Types
- Unary: restrict single variable
- Can be rolled into domain
- Why even have them?
- Binary: restricts two variables
- Global: restrict “all” variables
Assignments
- Assignments must be to values in each variable’s domain
- Assignment violates constraints?
- All variables assigned?
Four-Colorings
Two possibilities:
Graph Representations
- Constraint graph:
- Nodes are variables
- Edges are constraints
- Constraint hypergraph:
- Variables are nodes
- Constraints are nodes
- Edges show relationship
Why have two different representations?
Graph Representation I
Constraint graph: edges are constraints
Graph Representation II
Constraint hypergraph: constraints are nodes
Inference
- Constraints on one variable restrict others:
- \(X_1 \in \{A, B, C, D\}\) and \(X_2 \in \{A\}\)
- \(X_1 \neq X_2\)
- Inference: \(X_1 \in \{B, C, D\}\)
- If an unassigned variable has no domain…
Inference
- Arc consistency
- Reduce domains for pairs of variables
- Path consistency
- Assignment to two variables
- Reduce domain of third variable
Ordering
- Select-Unassgined-Variable(\(CSP, assignment\))
- Choose most-constrained variable1
- Order-Domain-Variables(\(CSP, var, assignment\))
- Why?
Restructuring
Tree-structured CSPs:
Logic
- Propositional symbols
- Similar to boolean variables
- Either True or False
- Represent something in “real world”
Sentences
- What is a linguistic sentence?
- Subject(s)
- Verb(s)
- Object(s)
- Relationships
- What is a logical sentence?
Familiar Logical Operators
- \(\neg\)
- “Not” operator, same as CS (
!
, not
, etc.)
- \(\land\)
- “And” operator, same as CS (
&&
, and
, etc.)
- This is sometimes called a conjunction.
- \(\lor\)
- “Inclusive Or” operator, same as CS.
- This is sometimes called a disjunction.
Unfamiliar Logical Operators
- \(\Rightarrow\)
- Logical implication.
- If \(X_0 \Rightarrow X_1\), \(X_1\) is always True when \(X_0\) is True.
- If \(X_0\) is False, the value of \(X_1\) is not constrained.
- \(\iff\)
- “If and only If.”
- If \(X_0 \iff X_1\), \(X_0\) and \(X_1\) are either both True or both False.
- Also called a biconditional.
Equivalent Statements
- \(X_0 \Rightarrow X_1\) alternatively:
- \((X_0 \land X_1) \lor \neg X_0\)
- \(X_0 \iff X_1\) alternatively:
- \((X_0 \land X_1) \lor (\neg X_0 \land \neg X_1)\)
Entailment
- \(KB \models A\)
- “Knowledge Base entails A”
- For every model in which \(KB\) is True, \(A\) is also True
- One-way relationship: \(A\) can be True for models where \(KB\) is not True.
- Vocabulary: \(A\) is the query
Knowing Things
Falsehood:
- \(KB \models \neg A\)
- No model exists where \(KB\) is True and \(A\) is True
It is possible to not know things:1
- \(KB \nvdash A\)
- \(KB \nvdash \neg A\)
Satisfiability
Commonly abbreviated “SAT”
First NP-complete problem
\((X_0 \land X_1) \lor X_2\)
- Satisfied by \(X_0 = \text{True}, X_1 = \text{False}, X_2 = \text{True}\)
- Satisfied for any \(X_0\) and \(X_1\) if \(X_2 = \text{True}\)
\(X_0 \land \neg X_0 \land X_1\)
- Cannot be satisfied by any values of \(X_0\) and \(X_1\)
Converting to CNF
- \(X_0 \iff X_1\)
- \((X_0 \Rightarrow X_1) \land (X_1 \Rightarrow X_0)\)
- \(X_0 \Rightarrow X_1\)
- \(\neg (X_0 \land X_1)\)
- \(\neg X_0 \lor \neg X_1\)
- \(\neg (X_0 \lor X_1)\)
- \(\neg X_0 \land \neg X_1\)
Probability
😌
- Very important, however
- Basis for remainder of course
- Sorry.